I tried to write a FB version of this tutorial.
https://medium.com/@model_train/video-t ... a194aeab44
I even used MSPAINT to rough out some graphics to go with it, but when nearly completed decided I was going about it the wrong way.
It seems to me you need to first work out how to write a data base of the "world". Then you work out the routines to manipulate that data base. Lastly you can work on the parser to make it easier call the required routines.
It is in fact the parser that interests me most, the problem of converting a human language sentence into meaningful actions, but first you need a "world" (data base) to test it on. However my weekend effort was going to start simple with single word commands like quit, north, south, east, west, look, pick_up, put_down, eat, open, close and so on ... or verb noun commands like go north, move north, and so on...
The code below was as far as I got. It only uses the commands quit, north, south, east, west, and look. Unlike the internet example my locations are east or west of each other. So at the start the command "east" will move the player from the lake location to the forest path location.
Any comments welcome.
To use the graphics with the code you need to convert the locations.png to locations.bmp
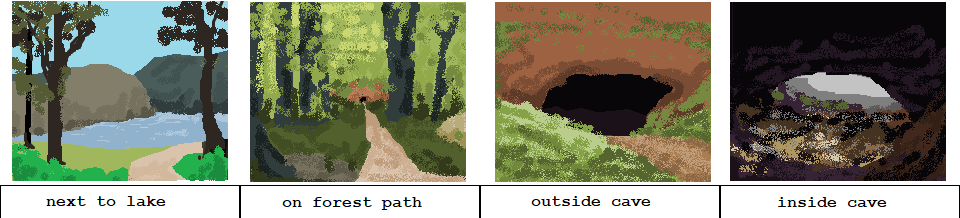
Code: Select all
' https://medium.com/@model_train/video-tutorial-create-a-text-adventure-game-in-30-minutes-in-the-browser-f4a194aeab44
screenres 960,500,32
'bload "locations.bmp"
dim shared as string sentence 'input sentence
dim shared as string words(0 to 20) 'list of words in sentence
dim shared as integer wordCount 'number of words in sentence
sub getWords()
dim As Integer charCount
dim as string word,char
wordCount = 0
charCount = 1
word = ""
while charCount < len(sentence)+1
word = "" 'next word
char = mid(sentence,charCount,1) 'get first character
'skip any leading spaces
while char = " " and charCount < len(sentence)+1
charCount = charCount + 1
char = mid(sentence,charCount,1) 'get next character
wend
'collect any word
char = mid(sentence,charCount,1)
while char <> " " and charCount < len(sentence)+1
word=word+char
charCount = charCount + 1
char = mid(sentence,charCount,1)
wend
words(wordCount)=word 'add word to word list
wordCount = wordCount + 1
wend
end sub
type ITEM
as string iName 'key, chest, apple, gem, ...
as integer state
as string states(1 to 2)
as integer location 'where the item resides
end type
dim shared as ITEM items(1 to 2)
items(1).iName = "TROLL"
items(1).states(1) = "alive"
items(1).states(2) = "asleep"
items(1).state = 1
items(1).location = 3 'outside cave
items(2).iName = "apple"
items(2).states(1) = "not eaten"
items(2).states(2) = "eaten"
items(2).state = 1
items(2).location = 3 'outside cave
items(3).iName = "treasure"
items(3).states(1) = "open"
items(3).states(2) = "closed"
items(3).state = 1
items(3).location = 4 'in cave
type LOCATION
as string description
'direction holds the location number that taking that direction will lead to
as integer direction(1 to 4) 'north, east, south, west POINTER INDEX to another location
as integer itemPtr(1 to 4) 'ptr to items at this location
end type
type AGENT
as string agentName '
as integer direction 'direction agent is looking
as integer location 'location of agent
end type
dim shared as AGENT player
player.direction = 2 'east
player.location = 1 'at the lake
dim shared as LOCATION locations(1 to 4)
locations(3).itemPtr(1) = 1 'point to TROLL item
locations(3).itemPtr(2) = 2 'point to apple item
locations(4).itemPtr(1) = 3 'point to treasure item
locations(1).description = "NEXT TO A LAKE"
locations(2).description = "ON FOREST PATH"
locations(3).description = "OUTSIDE CAVE"
locations(4).description = "INSIDE CAVE"
'next to lake = location 1
locations(1).direction(1)= 0 'north blocked
locations(1).direction(2)= 2 'east to forest path
locations(1).direction(3)= 0 'south blocked
locations(1).direction(4)= 0 'west blocked
'on forest path = location 2
locations(2).direction(1)= 0
locations(2).direction(2)= 3 'east to outside cave
locations(2).direction(3)= 0
locations(2).direction(4)= 1 'west to next to lake
'outside cave = location 3
locations(3).direction(1)= 0
locations(3).direction(2)= 4 'east to inside cave
locations(3).direction(3)= 0
locations(3).direction(4)= 2 'west to forest path
'inside cave = location 4
locations(4).direction(1)= 0
locations(4).direction(2)= 0
locations(4).direction(3)= 0
locations(4).direction(4)= 3 'west to outside cave
dim as string action
do
screenlock
cls
bload "locations.bmp"
locate 280\8,1
print
color rgb(100,100,255)
print " YOU ARE ";locations(player.location).description
color rgb(255,255,255)
print
print " Enter one of these single word commands"
print
print " +========+===================================+"
print " |COMMAND | action taken |"
print " +========+===================================|"
print " | quit | exits the program |"
print " +--------+-----------------------------------|"
print " | north | moves player to another location |"
print " +--------+-----------------------------------|"
print " | east | moves player to another location |"
print " +--------+-----------------------------------|"
print " | south | moves player to another location |"
print " +--------+-----------------------------------|"
print " | west | moves player to another location |"
print " +--------+-----------------------------------|"
print " | look | print location description |"
print " +--------+-----------------------------------+"
print
line ( (player.location-1)*240+10,0)-( (player.location-1)*240+10+217,180),rgb(255,0,0),b
line ( (player.location-1)*240+10-1,0)-( (player.location-1)*240+10+217-1,180),rgb(255,0,0),b
screenunlock
print
input "Type command:";sentence
print
cls
getWords()
action = words(0) 'only using first word from sentence
'change direction
if action ="north" then
player.direction = 1
end if
if action ="east" then
player.direction = 2
end if
if action ="south" then
player.direction = 3
end if
if action ="west" then
player.direction = 4
end if
'if move possible then change player's location
if locations(player.location).direction(player.direction) <> 0 then 'can move
player.location = locations(player.location).direction(player.direction) 'change location
else
color rgb(255,0,0)
print
print " DIRECTION BLOCKED"
end if
if action = "look" then
print
print " items at player's location"
color rgb(100,255,100)
for j as integer = 1 to 4
if locations(player.location).itemPtr(j) <> 0 then
print items(locations(player.location).itemPtr(j)).iName
end if
next j
color rgb(255,255,255)
print
end if
sleep 2
loop until action = "quit"
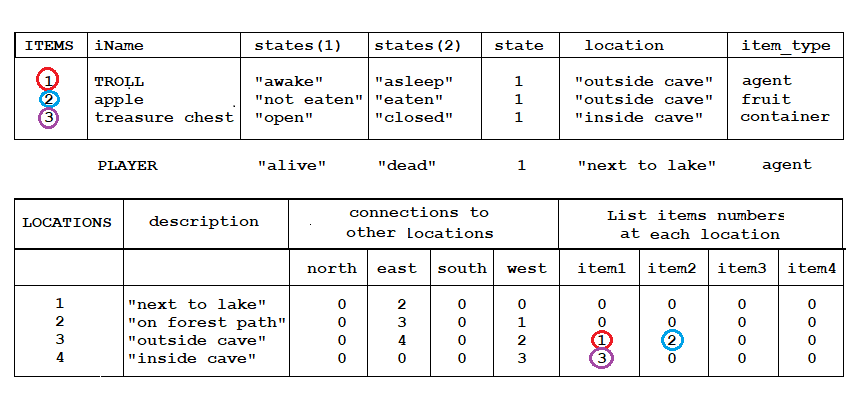