A simple program: Sum of two numbers
-
- Posts: 12
- Joined: Dec 29, 2006 10:45
A simple program: Sum of two numbers
Please,
How to do a GUI program with two labels and two
textfields (one for each number) to add two numbers
e put result in another field.
How to do a GUI program with two labels and two
textfields (one for each number) to add two numbers
e put result in another field.
-
- Posts: 3954
- Joined: Jan 01, 2009 7:03
- Location: Australia
Re: A simple program: Sum of two numbers
FreeBasic doesn't have GUI objects you have to learn how to use one of the GUI libraries.
-
- Posts: 8631
- Joined: May 28, 2005 3:28
- Contact:
Re: A simple program: Sum of two numbers
@Herminio Gomes you can try sGUI or GTK+ and here are an example made with my FLTK GUI wrapper.
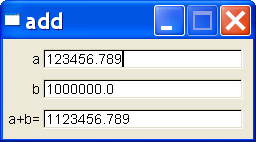
file: add.bas
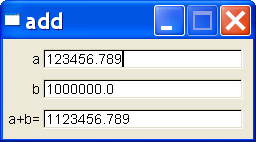
file: add.bas
Code: Select all
#include once "fltk-c.bi"
sub InputCB cdecl (byval self as FL_WIDGET ptr,byval arg as any ptr)
dim as Fl_Input ptr ptr in = arg
dim as double a = val(*Fl_Input_GetValue(in[0]))
dim as double b = val(*Fl_Input_GetValue(in[1]))
Fl_Input_SetValue in[2], str(a+b)
end sub
'
' main
'
var win = Fl_WindowNew(250,100,"add")
dim as any ptr in(...) = {Fl_Float_InputNew(40, 10, 200, 20,"a"), _
Fl_Float_InputNew(40, 40, 200, 20,"b"), _
Fl_Float_InputNew(40, 70, 200, 20,"a+b=") }
Fl_WidgetSetCallbackArg in(0),@InputCB,@in(0)
Fl_WidgetSetCallbackArg in(1),@InputCB,@in(0)
Fl_Input_SetReadonly in(2),1
Fl_WindowShow win
Fl_Run
Last edited by D.J.Peters on Oct 03, 2017 4:19, edited 1 time in total.
Re: A simple program: Sum of two numbers
Hello Herminio,
here's a GTK+ example, cross-platform as fltk. The output looks like
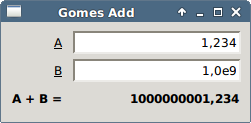
The window auto-adapts its context in case of a size change, which is important when you code for different devices (ie. PC, Beaglebone, Android, ...)
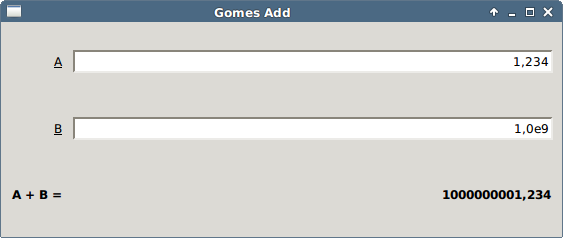
The labels have accelerators, so ie. when you press <Alternate>-<B> the cursor jumps in to the B entry. The code is prepared for I18N and L10N, so you can translate labels or tooltips in different languages, and also adapt the numbers format. (I use German formating, so the decimal separator is ',' instead of '.' here.)
Working with GTK+ is different from other toolkits. The only code I wrote is
which computes and sets the output. All the other code gets generated by tools
And the features of GTK+ are overhelming for beginners. But most of them are important (indispensable) for professional projects.
I enjoy that GTK+ is not only a GUI toolkit (with full UTF-8 support). It also provides a lot of helpful features in GLib, GObject and GIO. Ie. linked lists, trees, regular expressions, ... And the cairo grafics lib (which is part of GTK+) is very powerfull for 2D grafic output (screen and files, PNG pixel format or vector formats PDF, PS or SVG).
A lot of other high-level libraries are based on GTK+ (check https://developer.gnome.org/references for a complete list), ie.
Here's the source code
The GUI file gomes.ui (created by Glade3)
And here's the FB code (three files auto-generated by GladeToBac)
1.) gomes.bas (uses new 64 bit headers from GNOME header set)
2.) tobac/gomes_tobac.bas
3.) tobac/on_entry_changed.bas
here's a GTK+ example, cross-platform as fltk. The output looks like
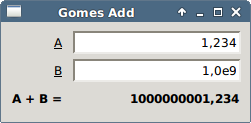
The window auto-adapts its context in case of a size change, which is important when you code for different devices (ie. PC, Beaglebone, Android, ...)
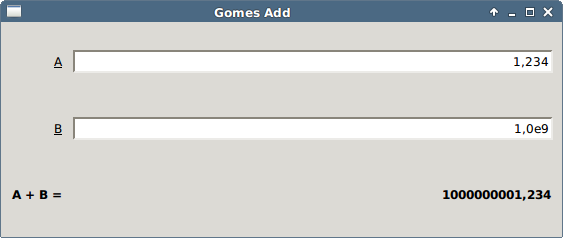
The labels have accelerators, so ie. when you press <Alternate>-<B> the cursor jumps in to the B entry. The code is prepared for I18N and L10N, so you can translate labels or tooltips in different languages, and also adapt the numbers format. (I use German formating, so the decimal separator is ',' instead of '.' here.)
Working with GTK+ is different from other toolkits. The only code I wrote is
Code: Select all
VAR res = _
VAL(*gtk_entry_get_text(GTK_ENTRY(GUI.entryA))) _
+ VAL(*gtk_entry_get_text(GTK_ENTRY(GUI.entryB)))
gtk_label_set_text(user_data, STR(res))
- 1. The grafical designer Glade3 is used to create the GUI (saved as gomes.ui).
2. File gomes.ui is loaded in to GladeToBac to auto-create the FB source files.
3. The above code lines are filled in to file tobac/on_entry_changed.bas (created by GladeToBac).
4. Two #ERROR lines (remainders) are commented and the main code gomes.bas gets compiled.
And the features of GTK+ are overhelming for beginners. But most of them are important (indispensable) for professional projects.
I enjoy that GTK+ is not only a GUI toolkit (with full UTF-8 support). It also provides a lot of helpful features in GLib, GObject and GIO. Ie. linked lists, trees, regular expressions, ... And the cairo grafics lib (which is part of GTK+) is very powerfull for 2D grafic output (screen and files, PNG pixel format or vector formats PDF, PS or SVG).
A lot of other high-level libraries are based on GTK+ (check https://developer.gnome.org/references for a complete list), ie.
- libgdaui for database access (SqLite, MySql, PostgreSql, ...)
- libgoocanvas for canvas widgets
- ...
Here's the source code
The GUI file gomes.ui (created by Glade3)
Code: Select all
<?xml version="1.0" encoding="UTF-8"?>
<!-- Generated with glade 3.16.1 -->
<interface>
<requires lib="gtk+" version="3.10"/>
<object class="GtkWindow" id="winMain">
<property name="can_focus">False</property>
<property name="hexpand">True</property>
<property name="vexpand">True</property>
<property name="title" translatable="yes">Gomes Add</property>
<property name="gravity">center</property>
<signal name="destroy" handler="gtk_main_quit" swapped="no"/>
<child>
<object class="GtkGrid" id="grid1">
<property name="visible">True</property>
<property name="can_focus">False</property>
<property name="hexpand">True</property>
<property name="vexpand">True</property>
<property name="border_width">9</property>
<property name="row_spacing">5</property>
<property name="column_spacing">9</property>
<property name="row_homogeneous">True</property>
<child>
<object class="GtkAccelLabel" id="label3">
<property name="visible">True</property>
<property name="can_focus">False</property>
<property name="xalign">1</property>
<property name="label" translatable="yes">A + B =</property>
<attributes>
<attribute name="weight" value="bold"/>
</attributes>
</object>
<packing>
<property name="left_attach">0</property>
<property name="top_attach">2</property>
<property name="width">1</property>
<property name="height">1</property>
</packing>
</child>
<child>
<object class="GtkAccelLabel" id="label2">
<property name="visible">True</property>
<property name="can_focus">False</property>
<property name="xalign">1</property>
<property name="label" translatable="yes">_B</property>
<property name="use_underline">True</property>
<property name="justify">right</property>
<property name="mnemonic_widget">entryB</property>
</object>
<packing>
<property name="left_attach">0</property>
<property name="top_attach">1</property>
<property name="width">1</property>
<property name="height">1</property>
</packing>
</child>
<child>
<object class="GtkAccelLabel" id="label1">
<property name="visible">True</property>
<property name="can_focus">False</property>
<property name="xalign">1</property>
<property name="label" translatable="yes">_A</property>
<property name="use_underline">True</property>
<property name="mnemonic_widget">entryA</property>
</object>
<packing>
<property name="left_attach">0</property>
<property name="top_attach">0</property>
<property name="width">1</property>
<property name="height">1</property>
</packing>
</child>
<child>
<object class="GtkEntry" id="entryB">
<property name="visible">True</property>
<property name="can_focus">True</property>
<property name="hexpand">True</property>
<property name="max_length">20</property>
<property name="width_chars">20</property>
<property name="xalign">1</property>
<property name="truncate_multiline">True</property>
<property name="caps_lock_warning">False</property>
<property name="primary_icon_tooltip_text" translatable="yes">Enter number B here</property>
<signal name="changed" handler="on_entry_changed" object="labelRes" swapped="no"/>
</object>
<packing>
<property name="left_attach">1</property>
<property name="top_attach">1</property>
<property name="width">1</property>
<property name="height">1</property>
</packing>
</child>
<child>
<object class="GtkEntry" id="entryA">
<property name="visible">True</property>
<property name="can_focus">True</property>
<property name="has_focus">True</property>
<property name="is_focus">True</property>
<property name="hexpand">True</property>
<property name="max_length">20</property>
<property name="width_chars">20</property>
<property name="xalign">1</property>
<property name="truncate_multiline">True</property>
<property name="caps_lock_warning">False</property>
<property name="primary_icon_tooltip_text" translatable="yes">Enter number A here</property>
<signal name="changed" handler="on_entry_changed" object="labelRes" swapped="no"/>
</object>
<packing>
<property name="left_attach">1</property>
<property name="top_attach">0</property>
<property name="width">1</property>
<property name="height">1</property>
</packing>
</child>
<child>
<object class="GtkLabel" id="labelRes">
<property name="visible">True</property>
<property name="can_focus">False</property>
<property name="xalign">1</property>
<property name="label" translatable="yes">0</property>
<attributes>
<attribute name="weight" value="bold"/>
</attributes>
</object>
<packing>
<property name="left_attach">1</property>
<property name="top_attach">2</property>
<property name="width">1</property>
<property name="height">1</property>
</packing>
</child>
</object>
</child>
</object>
</interface>
1.) gomes.bas (uses new 64 bit headers from GNOME header set)
Code: Select all
' ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
'< main program generated by utility GladeToBac V3.4 >
'< Hauptprogramm erzeugt von >
'< Generated at / Generierung am 2015-01-04, 09:38 >
' -----------------------------------------------------------------------------
'< Program info: >
CONST PROJ_NAME = "gomes" ' >
CONST PROJ_DESC = "GUI mini example" ' >
CONST PROJ_VERS = "0.0" ' >
CONST PROJ_YEAR = "2015" ' >
CONST PROJ_AUTH = "TJF" ' >
CONST PROJ_MAIL = "Thomas.Freiherr@gmx.net" ' >
CONST PROJ_WEBS = "" ' >
CONST PROJ_LICE = "GNU General Public License v3" ' >
'< >
'< Description / Beschreibung: >
'< >
'< >
'< License / Lizenz: >
'< >
'< This program is free software: you can redistribute it and/or modify >
'< it under the terms of the GNU General Public License as published by >
'< the Free Software Foundation, either version 3 of the License, or >
'< (at your option) any later version. >
'< >
'< This program is distributed in the hope that it will be useful, but >
'< WITHOUT ANY WARRANTY; without even the implied warranty of >
'< MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU >
'< General Public License for more details. >
'< >
'< You should have received a copy of the GNU General Public License along >
'< with this program. If not, see <http://www.gnu.org/licenses/>. >
'< >
' -----------------------------------------------------------------------------
'< Please prefer GNU GENERAL PUBLIC LICENSE to support open software. >
'< For more information please visit: http://www.fsf.org >
'< >
'< Bitte bevorzugen Sie die GNU GENERAL PUBLIC LICENSE und >
'< unterstuetzen Sie mit Ihrem Programm die freie Software >
'< Mehr Informationen finden Sie unter: http://www.fsf.org >
' -----------------------------------------------------------------------------
'< GladeToBac: general init / Allgemeine Initialisierungen >
#INCLUDE "Gir/Gtk-3.0.bi" ' GTK+library / GTK+ Bibliothek >
gtk_init(@__FB_ARGC__, @__FB_ARGV__) ' start GKT / GTK starten >
#INCLUDE "libintl.bi" ' load lib / Lib laden >
bindtextdomain(PROJ_NAME, EXEPATH & "/locale") ' path / Pfad >
bind_textdomain_codeset(PROJ_NAME, "UTF-8") ' set encoding / Zeichensatz >
textdomain(PROJ_NAME) ' Filename / Dateiname >
'< GladeToBac: end block / Blockende >
' vvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvv
' place your source code here / eigenen Quelltext hier einfuegen
'#ERROR GladeToBac: Quelltext einfügen!
' ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
'< GladeToBac: load GTK stuff / GTK Anbindung >
#INCLUDE "tobac/gomes_tobac.bas" ' Signale & GUI-XML >
'< GladeToBac: end block / Blockende >
' vvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvv
' here widgets are known, place your source code here
' hier sind die Widgets bekannt, eigenen Quelltext einfuegen
'#ERROR GladeToBac: Quelltext einfügen!
' ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
'< GladeToBac: run GTK main, then end / GTK Hauptschleife, dann Ende >
gtk_builder_connect_signals(GUI.XML, 0) ' Signale anbinden >
gtk_widget_show_all(GTK_WIDGET(GUI.winMain)) ' Hauptfenster darstellen >
gtk_main() ' main loop / Hauptschleife >
g_object_unref(GUI.XML) ' dereference / Referenz abbauen >
'< GladeToBac: end block / Blockende >
' vvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvv
' you may free memory and close files here
' ggf. hier Speicher freigeben und Dateien schliessen
Code: Select all
' ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
'< _tobac.bas modul generated by utility GladeToBac V3.4 >
'< Modul _tobac.bas erzeugt von >
'< Generated at / Generierung am 2015-01-04, 12:29 >
' -----------------------------------------------------------------------------
'< Main/Haupt Program name: gomes.bas >
'< Author: TJF >
'< Email: Thomas.Freiherr@gmx.net >
' -----------------------------------------------------------------------------
'< declare names, signal handlers, load GUI-XML do not edit! >
'< deklariert Namen, Signale, laedt GUI-XML nicht veraendern! >
' vvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvv
SCOPE
VAR er = gtk_check_version(3, 0, 0)
IF er THEN
?"Fehler/Error (GTK-Version):"
?*er
END 1
END IF
END SCOPE
TYPE GUIData
AS GtkBuilder PTR XML
AS GObject PTR _
winMain, entryB, entryA, labelRes
END TYPE
DIM SHARED AS GUIData GUI
GUI.XML = gtk_builder_new()
SCOPE
DIM AS GError PTR meld
IF 0 = gtk_builder_add_from_file(GUI.XML, "gomes.ui", @meld) THEN
WITH *meld
?"Fehler/Error (GTK-Builder):"
?*.message
END WITH
g_error_free(meld)
END 2
END IF
END SCOPE
WITH GUI
.winMain = gtk_builder_get_object(.XML, "winMain")
.entryB = gtk_builder_get_object(.XML, "entryB")
.entryA = gtk_builder_get_object(.XML, "entryA")
.labelRes = gtk_builder_get_object(.XML, "labelRes")
END WITH
#INCLUDE "on_entry_changed.bas"
Code: Select all
' ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
'< Signal handler generated by utility GladeToBac V3.4 >
'< Signal-Modul erzeugt von >
'< Generated at / Generierung am 2015-01-04, 10:16 >
' -----------------------------------------------------------------------------
'< Main/Haupt Program name: gui.bas >
'< Author: TJF >
'< Email: Thomas.Freiherr@gmx.net >
' -----------------------------------------------------------------------------
'< callback SUB/FUNCTION insert code! >
'< Ereignis Unterprogramm/Funktion Quelltext einfuegen! >
' vvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvvv
SUB on_entry_changed CDECL ALIAS "on_entry_changed" ( _
BYVAL widget AS GtkWidget PTR, _
BYVAL user_data AS gpointer) EXPORT ' Standard-Parameterliste
VAR res = _
VAL(*gtk_entry_get_text(GTK_ENTRY(GUI.entryA))) _
+ VAL(*gtk_entry_get_text(GTK_ENTRY(GUI.entryB)))
gtk_label_set_text(user_data, STR(res))
END SUB
-
- Posts: 491
- Joined: Sep 28, 2013 15:08
- Location: Germany
Re: A simple program: Sum of two numbers
Herminio,
what is your operating system? If you want to work on Windows GUI projects with FreeBasic in a comfortable manner similiar to Visual Basic with a mighty form designer, you should try FireFly (http://www.freebasic.net/forum/viewtopi ... =8&t=15796. WxFBE (http://www.freebasic.net/forum/viewtopi ... =8&t=20284) is an IDE with a simple form designer for Windows and Linux which uses actually WX-C or FLTK.
what is your operating system? If you want to work on Windows GUI projects with FreeBasic in a comfortable manner similiar to Visual Basic with a mighty form designer, you should try FireFly (http://www.freebasic.net/forum/viewtopi ... =8&t=15796. WxFBE (http://www.freebasic.net/forum/viewtopi ... =8&t=20284) is an IDE with a simple form designer for Windows and Linux which uses actually WX-C or FLTK.
Re: A simple program: Sum of two numbers
As a Windows program this is quite easily coded:
Code: Select all
#include "windows.bi"
shell "title CHECK THE ADDITION" 'print answer to the console as a check
declare sub ADDUP()
dim shared as hwnd b1,b2,answer
const MainWindow ="#32770"
Dim As MSG msg
var hWnd=CreateWindowEx( 0,MainWindow, "ADD UP", WS_OVERLAPPEDWINDOW Or WS_VISIBLE, 200, 200, 500, 600, 0, 0, 0, 0 )
B1 = CreateWindowEx( 0, "EDIT", "", ws_border Or WS_VISIBLE Or WS_CHILD , 20, 80, 450, 30, hWnd, 0, 0, 0 )
B2 = CreateWindowEx( 0, "EDIT", "", ws_border Or WS_VISIBLE Or WS_CHILD , 20, 200, 450, 30, hWnd, 0, 0, 0 )
answer = CreateWindowEx( 0, "EDIT", "", ws_border Or WS_VISIBLE Or WS_CHILD Or WS_HSCROLL Or ES_AUTOHSCROLL Or ES_READONLY, 20, 400, 450, 50, hWnd, 0, 0, 0 )
var label=CreateWindowEx( 0, "STATIC", "ANSWER:",WS_VISIBLE Or WS_CHILD , 20, 370, 450, 30, hWnd, 0, 0, 0 )
var ADDBUTTON= CreateWindowEx( 0,"BUTTON", "ADD", WS_VISIBLE Or WS_CHILD, 20, 20 , 70, 30, hWnd, 0, 0, 0 )
While GetMessage( @msg, 0, 0, 0 )
TranslateMessage( @msg )
DispatchMessage( @msg )
Select Case msg.hwnd
Case hWnd
Select Case msg.message
Case 273
End
End Select
'__________________
Case AddButton
select case msg.message
Case WM_LBUTTONDOWN
ADDUP
end select
end select
wend
sub ADDUP()
dim as string outtext1,outtext2
dim as integer charcount1,charcount2
charcount1 = GetWindowTextLength(B1)
charcount2 = GetWindowTextLength(B2)
outtext1 = string(charcount1," ")
outtext2 = string(charcount2," ")
GetWindowText(B1,outtext1,charcount1+1)
GetWindowText(B2,outtext2,charcount2+1)
shell "echo " & val(outtext1)+val(outtext2)'print answer to the console as a check
var result= str(val(outtext1)+val(outtext2))
setWindowText(answer,result)
end sub
-
- Posts: 3954
- Joined: Jan 01, 2009 7:03
- Location: Australia
Re: A simple program: Sum of two numbers
Well it is only easily coded if you happen to understand the WindowAPI.dodicat wrote:As a Windows program this is quite easily coded:
Compare your example with an identical program written using Visual Basic and then tell me which is easily coded.
The whole advantage of high level languages to the casual programmer is they can write readable code that does exactly the same thing.
Re: A simple program: Sum of two numbers
Well, a form designer is a different ball game.
Did you find TJF's method easier than my 45 code lines?
Or, indeed, D.J.Peter's method?
The Win api is included with the FB distro (32 bit anyhow), so why not use it for simple programs?
Did you find TJF's method easier than my 45 code lines?
Or, indeed, D.J.Peter's method?
The Win api is included with the FB distro (32 bit anyhow), so why not use it for simple programs?
-
- Posts: 3954
- Joined: Jan 01, 2009 7:03
- Location: Australia
Re: A simple program: Sum of two numbers
Perhaps Java would have been a better example?dodicat wrote:Well, a form designer is a different ball game.
In a BASIC like program all you should need is,
dim as TextBox tbNumber1("",100,30 etc)
And an event loop with the code to deal with each possible event.
My comments would apply to them as well. Their code is not immediately readable.Did you find TJF's method easier than my 45 code lines?
Or, indeed, D.J.Peter's method?
Cryptic code is the same whatever language is used to write it in and defeats the whole beauty of BASIC like code.
The only reason not to use the Win api I guess is if you want it to run on linux?The Win api is included with the FB distro (32 bit anyhow), so why not use it for simple programs?
Re: A simple program: Sum of two numbers
Createwindowex gives the main window, textboxes "EDIT", labels "STATIC", e.t.c.
Only thing is there are quite a few parameters, this makes it general but ugly code.
But once you have created your main window and the child windows on it, then the loop can be very simple.
Here is a simplified example of ADD:
The forum format makes it look terrible, but pasted to fbide it looks better.
Only thing is there are quite a few parameters, this makes it general but ugly code.
But once you have created your main window and the child windows on it, then the loop can be very simple.
Here is a simplified example of ADD:
Code: Select all
#include "windows.bi"
dim shared as HWND Input1,Input2,answer 'shared, used in sub addup()
const MainWindow ="#32770" 'always use this number
Dim As MSG msg
var MAINWIN=CreateWindowEx(0,MainWindow,"ADD UP", WS_OVERLAPPEDWINDOW Or WS_VISIBLE,200,200,500,600,0,0,0,0)
Input1 = CreateWindowEx(0,"EDIT","0",WS_BORDER Or WS_VISIBLE Or WS_CHILD,20,80,450,30,MAINWIN,0,0,0)
Input2 = CreateWindowEx(0,"EDIT","0",WS_BORDER Or WS_VISIBLE Or WS_CHILD,20,200,450,30,MAINWIN,0,0,0)
answer = CreateWindowEx(0,"EDIT"," ",WS_BORDER Or WS_VISIBLE Or WS_CHILD Or ES_READONLY,20,400,450,30,MAINWIN,0,0,0)
var label = CreateWindowEx(0,"STATIC","ANSWER:",WS_VISIBLE Or WS_CHILD,20,370,450,30,MAINWIN,0,0,0)
sub ADDUP()
dim as string outtext1,outtext2
dim as integer charcount1,charcount2
static as string last1,last2
charcount1 = GetWindowTextLength(Input1)
charcount2 = GetWindowTextLength(Input2)
outtext1 = string(charcount1," ")
outtext2 = string(charcount2," ")
GetWindowText(Input1,outtext1,charcount1+1)
GetWindowText(Input2,outtext2,charcount2+1)
if last1<>outtext1 or last2<>outtext2 then
var result= str(val(outtext1)+val(outtext2))
setWindowText(answer,result)
end if
last1=outtext1
last2=outtext2
end sub
FreeConsole
While GetMessage(@msg,0,0,0)
TranslateMessage(@msg)
DispatchMessage(@msg)
addup
if msg.message=273 then end
wend
-
- Posts: 3954
- Joined: Jan 01, 2009 7:03
- Location: Australia
Re: A simple program: Sum of two numbers
@dodicat
A very nice example. Years ago I remember learning (and now forgotten in detail) to create windows and their objects and print text strings and so on using C++ and Assembler source code.
http://win32assembly.programminghorizon ... rials.html
Can't remember offhand what C++ tutorials I used.
I think programmers can shift all the complex setups and default values into an include file to make the main code look cleaner?
However the bottom line is that I like to spend my spare time programming not learning to program or learning yet another language or operating system. I was spoiled by the ease of assembler programming on the older simpler machines like the C64 where you did not have to deal with made up high level API magic numbers to remember.
const MainWindow ="#32770" 'always use this number
There is no understanding involved in that line. You just place it in there as a magical incantation. You would never be able to figure it out yourself from any knowledge of hardware.
A very nice example. Years ago I remember learning (and now forgotten in detail) to create windows and their objects and print text strings and so on using C++ and Assembler source code.
http://win32assembly.programminghorizon ... rials.html
Can't remember offhand what C++ tutorials I used.
I think programmers can shift all the complex setups and default values into an include file to make the main code look cleaner?
However the bottom line is that I like to spend my spare time programming not learning to program or learning yet another language or operating system. I was spoiled by the ease of assembler programming on the older simpler machines like the C64 where you did not have to deal with made up high level API magic numbers to remember.
const MainWindow ="#32770" 'always use this number
There is no understanding involved in that line. You just place it in there as a magical incantation. You would never be able to figure it out yourself from any knowledge of hardware.
Re: A simple program: Sum of two numbers
Yes, indeed, IMHO writing 4 lines of code is easier than writing 45. Especialy when you add the lines to some existing code and everything has to be compatible with one another.dodicat wrote:Did you find TJF's method easier than my 45 code lines?
-
- Posts: 491
- Joined: Sep 28, 2013 15:08
- Location: Germany
Re: A simple program: Sum of two numbers
I have the same answer concerning D.J. Peter's example. Maybe the example is not so easy understandable for a beginner without more comments, but FLTK is really easy to understand and to use, see http://www.freebasic-portal.de/tutorial ... n-110.html.
WinAPI is rather complex, and it is difficult to see where to begin in the documentation. It may be helpful to use FBEdit and its form designer in order to generate the basic code for your window, but then you have to write your program with the aid of WinAPI. This can be avoided widely by using FireFly with its own well documented functions.
An easy to use library for Windows GUI is Vanya's Window9 (see http://www.freebasic.net/forum/viewtopi ... lit=FBGUI2. The code is very clear and compact and needs nearly no knowledge of WinAPI.
@Herminio, I hope you are not confused now. There are a lot of solutions for FreeBasic.
WinAPI is rather complex, and it is difficult to see where to begin in the documentation. It may be helpful to use FBEdit and its form designer in order to generate the basic code for your window, but then you have to write your program with the aid of WinAPI. This can be avoided widely by using FireFly with its own well documented functions.
An easy to use library for Windows GUI is Vanya's Window9 (see http://www.freebasic.net/forum/viewtopi ... lit=FBGUI2. The code is very clear and compact and needs nearly no knowledge of WinAPI.
@Herminio, I hope you are not confused now. There are a lot of solutions for FreeBasic.
-
- Posts: 252
- Joined: Mar 12, 2006 16:25
Re: A simple program: Sum of two numbers
Well the difference between Freebasic and ... is:
The most simple solution compiled with fbc -s console :
a slightly longer example with pure freebasic using graphics screen:
fbc -s gui
- The most simple solution with Java which is using GUI elements is more than twice as long.
- The most simple solution with Freebasic which is using GUI elements is more than twice as cryptical.
- The most simple solution with C++ which is using GUI elements is even more than twice as cryptical.
- Just use a good GUI Builder with the language / framework / ide of your choice - just as easy as the simple code above.
the true length of the code will be hidden from you. Be happy!
The most simple solution compiled with fbc -s console :
Code: Select all
Dim As String a,b
Do
Input "Zahl a: ",a
Input "Zahl b: ",b
If (Len(a) + Len(b)) = 0 Then Exit do
Print "a + b = ";Trim(Str(Val(a)+Val(b)))
Loop
fbc -s gui
Code: Select all
#Include "fbgfx.bi"
ScreenRes 200,120,32
Color 0,RGB(255, 255, 255)
Cls
Dim As String a,b
Do
Print
Input "Zahl a: ",a
Input "Zahl b: ",b
If (Len(a) + Len(b)) = 0 Then Exit do
Print "a + b = ";Trim(Str(Val(a)+Val(b)))
Loop
- The most simple solution with Freebasic which is using GUI elements is more than twice as cryptical.
- The most simple solution with C++ which is using GUI elements is even more than twice as cryptical.
- Just use a good GUI Builder with the language / framework / ide of your choice - just as easy as the simple code above.
the true length of the code will be hidden from you. Be happy!
-
- Posts: 491
- Joined: Sep 28, 2013 15:08
- Location: Germany
Re: A simple program: Sum of two numbers
"Just use a good GUI Builder ...":
Code with FireFly - 6 lines to be written by the user:
Code with FireFly - 6 lines to be written by the user:
Code: Select all
Function FORM1_COMMANDADD_BN_CLICKED ( _
ControlIndex As Integer, _ ' index in Control Array
hWndForm As Hwnd, _ ' handle of Form
hWndControl As Hwnd, _ ' handle of Control
idButtonControl As Integer _ ' identifier of button
) As Integer
'This code must be written by the user:
Dim As Double a, b, result
a = Val(Ff_Textbox_Gettext(HWND_FORM1_TEXTA))
b = Val(Ff_Textbox_Gettext(HWND_FORM1_TEXTb))
result = a + b
Ff_Textbox_Settext(HWND_FORM1_TEXTRESULT, Str(result))
Return 1
End Function